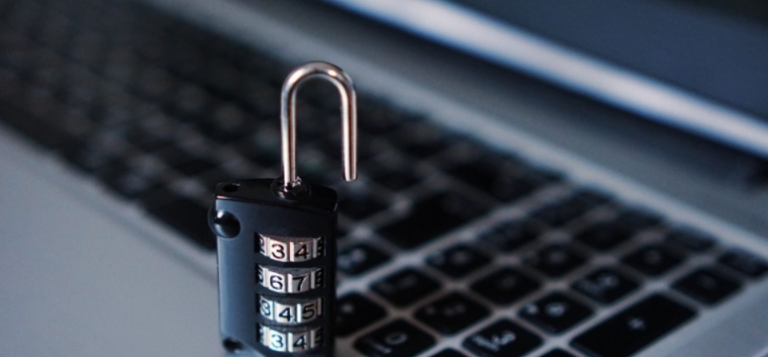
Gem of the Week: Figaro
September 16, 2016 Jamey Alea 1 Comment
So let’s say you’ve already got your app up and running with whatever service you need and a fancy gem to help you integrate it, like Stripe or Twilio. Great! But to set up the configurations, you need to use your API secret keys and you don’t want those accessible in your codebase or pushed to your repository. I mean, they’re suppose to be secret! Plus, maybe you have different keys for different Rails environments and you don’t want to hardcode them in. The solution is Environment Variables and there are a few ways you can set them up in your app. One good solution is the Figaro gem.
Adding Figaro to your gemfile and installing it will automatically generate your config/application.yml file and add it to your .gitignore file. Ensuring that this file is included in .gitignore is super important, because it will contain all your secret keys and excluding them from your repo is the whole point!
Let’s use Stripe as an example. In this previous article, I went over how to configure Stripe like this:
if Rails.env.production? Rails.configuration.stripe = { :publishable_key => "your_live_publishable_key", :secret_key => "your_live_secret_key" } else Rails.configuration.stripe = { :publishable_key => "your_test_publishable_key", :secret_key => "your_test_secret_key" } end
These are great examples of values that should be saved as environment variables. Let’s put them in the config/application.yml file:
stripe_publishable_key: "your_live_publishable_key" stripe_secret_key: "your_live_secret_key" development: stripe_publishable_key: "your_test_publishable_key" stripe_secret_key: "your_test_secret_key"
(Obviously, these should be the actual values of your API keys.) Now your app will know to use the correct values for the correct environments. It would be wise to set up a section for each environment in this file (and remember that .yml files are whitespace significant!)
Now, your Stripe configuration should look like this instead:
Rails.configuration.stripe = { :publishable_key => ENV[“stripe_publishable_key”], :secret_key => ENV[“stripe_secret_key”] }
Much cleaner, and your secrets are safe!
If you don’t specify a different value for another environment, it will default to the production value. But if you don’t want there to be a value at all, you can also remove variables from a certain environments by nullifying them out, like this:
stripe_publishable_key: "your_live_publishable_key" stripe_secret_key: "your_live_secret_key" test: stripe_publishable_key: ~ stripe_secret_key: ~
Now the test environment won’t have stripe keys and it won’t automatically default to the live keys.
Figaro also lets you require that certain keys have values set. If you create a config initializer file for Figaro, you can stipulate which keys should be required.
Figaro.require_keys(“stripe_publishable_key”, “stripe_secret_key”)
Now if these two keys aren’t set in the config/application.yml file, your app will throw errors when you try to boot up your server.
Check out the Figaro gem documentation for more info, particularly deployment instructions for Heroku which they make really easy.
(This article was originally published on Uptime.)
Comments